How To Store User Uploaded File Javascript
It's a known fact that file selection inputs are hard to way the way developers desire to, and so many simply hide it and create a button that opens the file pick dialog instead. Nowadays, though, we have an even fancier way of treatment file selection: drag and drop.
Technically, this was already possible because most (if non all) implementations of the file selection input allowed yous to elevate files over information technology to select them, simply this requires you to really show the file
element. And then, let's actually use the APIs given to u.s.a. by the browser to implement a elevate-and-driblet file selector and uploader.
In this article, we'll be using "vanilla" ES2015+ JavaScript (no frameworks or libraries) to consummate this projection, and it is assumed that y'all have a working cognition of JavaScript in the browser. This case — aside from the ES2015+ syntax, which can easily inverse to ES5 syntax or transpiled by Boom-boom — should be compatible with every evergreen browser plus IE 10 and eleven.
Here'southward a quick look at what you'll exist making:
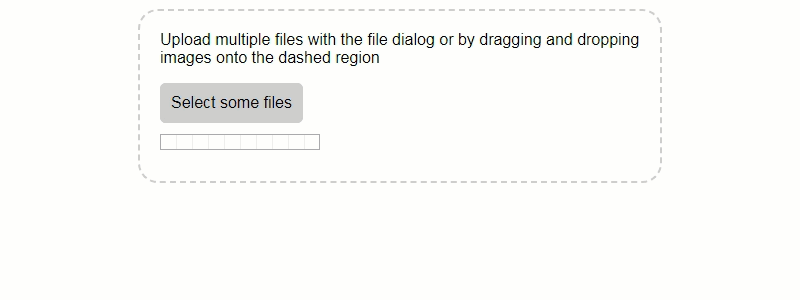
Elevate-and-Drop Events
The get-go matter we need to discuss is the events related to elevate-and-drib because they are the driving strength behind this characteristic. In all, there are eight events the browser fires related to drag and drop: drag
, dragend
, dragenter
, dragexit
, dragleave
, dragover
, dragstart
, and drop
. Nosotros won't be going over all of them because drag
, dragend
, dragexit
, and dragstart
are all fired on the element that is being dragged, and in our case, nosotros'll be dragging files in from our file system rather than DOM elements, so these events will never pop up.
If y'all're curious about them, you can read some documentation about these events on MDN.
More than after jump! Continue reading below ↓
As you might expect, you can register effect handlers for these events in the same mode you register event handlers for nigh browser events: via addEventListener
.
let dropArea = certificate.getElementById('drop-area') dropArea.addEventListener('dragenter', handlerFunction, false) dropArea.addEventListener('dragleave', handlerFunction, false) dropArea.addEventListener('dragover', handlerFunction, false) dropArea.addEventListener('drop', handlerFunction, imitation)
Here's a trivial tabular array describing what these events practise, using dropArea
from the code sample in order to make the language clearer:
Event | When Is It Fired? |
---|---|
dragenter | The dragged item is dragged over dropArea, making information technology the target for the drib consequence if the user drops it there. |
dragleave | The dragged item is dragged off of dropArea and onto another element, making it the target for the drib issue instead. |
dragover | Every few hundred milliseconds, while the dragged particular is over dropArea and is moving. |
drib | The user releases their mouse button, dropping the dragged item onto dropArea. |
Note that the dragged item is dragged over a kid of dropArea
, dragleave
will fire on dropArea
and dragenter
will fire on that child element because it is the new target
. The drop
upshot volition propagate up to dropArea
(unless propagation is stopped by a different result listener before it gets there), so information technology'll nonetheless fire on dropArea
despite information technology not existence the target
for the event.
Too note that in club to create custom drag-and-drop interactions, you'll need to phone call outcome.preventDefault()
in each of the listeners for these events. If you don't, the browser will end upwardly opening the file y'all dropped instead of sending it along to the drop
outcome handler.
Setting Up Our Form
Before we beginning adding drag-and-driblet functionality, we'll need a basic form with a standard file
input. Technically this isn't necessary, simply it's a expert thought to provide it as an alternative in case the user has a browser without back up for the drag-and-drop API.
<div id="driblet-area"> <form class="my-form"> <p>Upload multiple files with the file dialog or by dragging and dropping images onto the dashed region</p> <input type="file" id="fileElem" multiple accept="prototype/*" onchange="handleFiles(this.files)"> <characterization grade="button" for="fileElem">Select some files</label> </form> </div>
Pretty simple structure. You may observe an onchange
handler on the input
. We'll take a look at that later on. It would also be a good thought to add together an action
to the form
and a submit
push to help out those people who don't have JavaScript enabled. Then you tin apply JavaScript to get rid of them for a cleaner course. In any case, you will need a server-side script to accept the upload, whether it's something developed in-firm, or you're using a service like Cloudinary to do it for you. Other than those notes, at that place'due south nothing special hither, so allow's throw some styles in:
#driblet-area { border: 2px dashed #ccc; border-radius: 20px; width: 480px; font-family: sans-serif; margin: 100px auto; padding: 20px; } #driblet-surface area.highlight { border-color: majestic; } p { margin-peak: 0; } .my-form { margin-bottom: 10px; } #gallery { margin-top: 10px; } #gallery img { width: 150px; margin-bottom: 10px; margin-right: 10px; vertical-align: centre; } .button { display: inline-block; padding: 10px; background: #ccc; cursor: pointer; edge-radius: 5px; edge: 1px solid #ccc; } .push button:hover { background: #ddd; } #fileElem { display: none; }
Many of these styles aren't coming into play yet, but that's OK. The highlights, for now, are that the file
input is hidden, but its label
is styled to look like a push button, and then people will realize they can click it to bring up the file option dialog. We're too post-obit a convention past outlining the drop area with dashed lines.
Adding The Drag-and-Drop Functionality
Now we get to the meat of the situation: drag and drop. Permit'southward throw a script in at the lesser of the folio, or in a separate file, however y'all feel like doing it. The offset thing nosotros need in the script is a reference to the drib surface area so we can attach some events to it:
let dropArea = document.getElementById('driblet-area')
At present let'southward add some events. We'll start off with adding handlers to all the events to forestall default behaviors and finish the events from bubbling upwardly whatsoever higher than necessary:
;['dragenter', 'dragover', 'dragleave', 'drop'].forEach(eventName => { dropArea.addEventListener(eventName, preventDefaults, false) }) function preventDefaults (e) { east.preventDefault() east.stopPropagation() }
Now let'southward add together an indicator to permit the user know that they accept indeed dragged the item over the correct area by using CSS to change the color of the border color of the driblet surface area. The styles should already exist there under the #drop-area.highlight
selector, then allow'south employ JS to add and remove that highlight
grade when necessary.
;['dragenter', 'dragover'].forEach(eventName => { dropArea.addEventListener(eventName, highlight, imitation) }) ;['dragleave', 'driblet'].forEach(eventName => { dropArea.addEventListener(eventName, unhighlight, false) }) function highlight(e) { dropArea.classList.add('highlight') } office unhighlight(e) { dropArea.classList.remove('highlight') }
We had to use both dragenter
and dragover
for the highlighting because of what I mentioned earlier. If you start off hovering straight over dropArea
so hover over one of its children, then dragleave
will be fired and the highlight will be removed. The dragover
event is fired later on the dragenter
and dragleave
events, so the highlight volition exist added back onto dropArea
before we see it being removed.
We besides remove the highlight when the dragged item leaves the designated area or when you drib the item.
Now all we need to do is figure out what to do when some files are dropped:
dropArea.addEventListener('drop', handleDrop, simulated) function handleDrop(eastward) { allow dt = e.dataTransfer let files = dt.files handleFiles(files) }
This doesn't bring us anywhere near completion, but it does ii of import things:
- Demonstrates how to get the data for the files that were dropped.
- Gets us to the same place that the
file
input
was at with itsonchange
handler: waiting forhandleFiles
.
Keep in mind that files
is non an array, but a FileList
. And so, when we implement handleFiles
, nosotros'll need to catechumen it to an array in guild to iterate over it more easily:
function handleFiles(files) { ([...files]).forEach(uploadFile) }
That was anticlimactic. Let'due south get into uploadFile
for the real meaty stuff.
function uploadFile(file) { let url = 'YOUR URL Hither' let formData = new FormData() formData.append('file', file) fetch(url, { method: 'Mail', trunk: formData }) .and then(() => { /* Done. Inform the user */ }) .take hold of(() => { /* Error. Inform the user */ }) }
Hither we use FormData
, a born browser API for creating form information to transport to the server. We then apply the fetch
API to actually send the image to the server. Make certain you change the URL to work with your dorsum-end or service, and formData.suspend
whatever additional form data you may need to give the server all the information information technology needs. Alternatively, if you want to support Internet Explorer, you may want to use XMLHttpRequest
, which means uploadFile
would expect like this instead:
function uploadFile(file) { var url = 'YOUR URL HERE' var xhr = new XMLHttpRequest() var formData = new FormData() xhr.open('Mail service', url, true) xhr.addEventListener('readystatechange', role(e) { if (xhr.readyState == four && xhr.status == 200) { // Done. Inform the user } else if (xhr.readyState == 4 && xhr.status != 200) { // Error. Inform the user } }) formData.suspend('file', file) xhr.send(formData) }
Depending on how your server is fix up, you may want to check for different ranges of status
numbers rather than just 200
, but for our purposes, this will work.
Boosted Features
That is all of the base functionality, merely often nosotros desire more than functionality. Specifically, in this tutorial, we'll be adding a preview pane that displays all the chosen images to the user, then we'll add together a progress bar that lets the user see the progress of the uploads. Then, let'south become started with previewing images.
Image Preview
In that location are a couple of ways you lot could practice this: you could look until later on the paradigm has been uploaded and ask the server to send the URL of the image, but that means you need to await and images can be pretty large sometimes. The alternative — which we'll be exploring today — is to use the FileReader API on the file data nosotros received from the drib
event. This is asynchronous, and you could alternatively use FileReaderSync, but nosotros could be trying to read several large files in a row, and so this could block the thread for quite a while and really ruin the experience. And so allow's create a previewFile
part and see how it works:
function previewFile(file) { let reader = new FileReader() reader.readAsDataURL(file) reader.onloadend = function() { permit img = document.createElement('img') img.src = reader.effect document.getElementById('gallery').appendChild(img) } }
Here we create a new FileReader
and call readAsDataURL
on it with the File
object. As mentioned, this is asynchronous, so nosotros need to add an onloadend
event handler in order to get the result of the read. We then use the base of operations 64 data URL as the src
for a new image element and add together it to the gallery
element. In that location are only ii things that need to be done to make this work now: add the gallery
element, and brand certain previewFile
is really chosen.
Showtime, add the following HTML right afterward the end of the form
tag:
Nil special; it'due south just a div. The styles are already specified for it and the images in information technology, then at that place'due south cypher left to practice there. Now let's change the handleFiles
function to the following:
role handleFiles(files) { files = [...files] files.forEach(uploadFile) files.forEach(previewFile) }
At that place are a few ways you lot could take done this, such as composition, or a single callback to forEach
that ran uploadFile
and previewFile
in information technology, but this works too. And with that, when y'all drop or select some images, they should prove up almost instantly below the grade. The interesting thing about this is that — in certain applications — yous may not really want to upload images, just instead store the data URLs of them in localStorage
or another client-side cache to be accessed by the app later. I can't personally think of whatever good use cases for this, simply I'yard willing to bet in that location are some.
Tracking Progress
If something might accept a while, a progress bar can help a user realize progress is actually beingness fabricated and give an indication of how long it volition take to be completed. Calculation a progress indicator is pretty easy thanks to the HTML5 progress
tag. Let's start past adding that to the HTML lawmaking this time.
<progress id="progress-bar" max=100 value=0></progress>
You tin can plop that in right after the characterization
or between the class
and gallery div
, whichever you fancy more. For that thing, yous tin can place information technology wherever you want inside the torso
tags. No styles were added for this example, so it will prove the browser's default implementation, which is serviceable. Now permit's work on adding the JavaScript. Nosotros'll first look at the implementation using fetch
and then we'll show a version for XMLHttpRequest
. To start, we'll need a couple of new variables at the top of the script :
let filesDone = 0 let filesToDo = 0 permit progressBar = document.getElementById('progress-bar')
When using fetch
we're only able to determine when an upload is finished, and then the only information we track is how many files are selected to upload (as filesToDo
) and the number of files that accept finished uploading (equally filesDone
). We're likewise keeping a reference to the #progress-bar
chemical element so we can update it quickly. Now let's create a couple of functions for managing the progress:
part initializeProgress(numfiles) { progressBar.value = 0 filesDone = 0 filesToDo = numfiles } function progressDone() { filesDone++ progressBar.value = filesDone / filesToDo * 100 }
When nosotros start uploading, initializeProgress
will be called to reset the progress bar. Then, with each completed upload, we'll call progressDone
to increment the number of completed uploads and update the progress bar to show the electric current progress. And then let's call these functions by updating a couple of onetime functions:
role handleFiles(files) { files = [...files] initializeProgress(files.length) // <- Add this line files.forEach(uploadFile) files.forEach(previewFile) } role uploadFile(file) { permit url = 'YOUR URL Hither' permit formData = new FormData() formData.append('file', file) fetch(url, { method: 'Postal service', trunk: formData }) .then(progressDone) // <- Add `progressDone` call here .catch(() => { /* Error. Inform the user */ }) }
And that's it. Now let'south take a look at the XMLHttpRequest
implementation. We could simply make a quick update to uploadFile
, but XMLHttpRequest
actually gives us more functionality than fetch
, namely we're able to add an event listener for upload progress on each request, which will periodically give us information near how much of the asking is finished. Because of this, nosotros need to track the percentage completion of each request instead of just how many are done. And then, permit's get-go with replacing the declarations for filesDone
and filesToDo
with the following:
let uploadProgress = []
So we need to update our functions also. Nosotros'll rename progressDone
to updateProgress
and modify them to be the following:
function initializeProgress(numFiles) { progressBar.value = 0 uploadProgress = [] for(permit i = numFiles; i > 0; i--) { uploadProgress.push(0) } } function updateProgress(fileNumber, per centum) { uploadProgress[fileNumber] = percent permit total = uploadProgress.reduce((tot, curr) => tot + curr, 0) / uploadProgress.length progressBar.value = full }
Now initializeProgress
initializes an array with a length equal to numFiles
that is filled with zeroes, denoting that each file is 0% complete. In updateProgress
we discover out which paradigm is having their progress updated and change the value at that index to the provided percent
. We then calculate the full progress per centum by taking an boilerplate of all the percentages and update the progress bar to reflect the calculated full. We still call initializeProgress
in handleFiles
the same as nosotros did in the fetch
example, so now all nosotros need to update is uploadFile
to phone call updateProgress
.
function uploadFile(file, i) { // <- Add `i` parameter var url = 'YOUR URL HERE' var xhr = new XMLHttpRequest() var formData = new FormData() xhr.open('POST', url, truthful) // Add following upshot listener xhr.upload.addEventListener("progress", role(east) { updateProgress(i, (e.loaded * 100.0 / eastward.total) || 100) }) xhr.addEventListener('readystatechange', function(due east) { if (xhr.readyState == 4 && xhr.status == 200) { // Done. Inform the user } else if (xhr.readyState == 4 && xhr.status != 200) { // Error. Inform the user } }) formData.append('file', file) xhr.send(formData) }
The first thing to notation is that nosotros added an i
parameter. This is the index of the file in the list of files. We don't need to update handleFiles
to pass this parameter in because it is using forEach
, which already gives the index of the element as the 2nd parameter to callbacks. We also added the progress
issue listener to xhr.upload
so nosotros can telephone call updateProgress
with the progress. The event object (referred to as e
in the lawmaking) has two pertinent pieces of information on it: loaded
which contains the number of bytes that have been uploaded so far and total
which contains the number of bytes the file is in total.
The || 100
piece is in there because sometimes if there is an fault, e.loaded
and e.total
volition be cypher, which ways the calculation will come up out as NaN
, and so the 100
is used instead to report that the file is done. You could also employ 0
. In either instance, the error volition testify upwardly in the readystatechange
handler so that you can inform the user about them. This is merely to forbid exceptions from being thrown for trying to exercise math with NaN
.
Determination
That'due south the concluding piece. Yous now accept a spider web page where you tin upload images via drag and drop, preview the images being uploaded immediately, and see the progress of the upload in a progress bar. Yous can meet the last version (with XMLHttpRequest
) in action on CodePen, but be aware that the service I upload the files to has limits, so if a lot of people test it out, information technology may break for a time.
(rb, ra, il)
Source: https://www.smashingmagazine.com/2018/01/drag-drop-file-uploader-vanilla-js/
Posted by: selfridgehatilight1943.blogspot.com
0 Response to "How To Store User Uploaded File Javascript"
Post a Comment